JavaRanch Cattle Drive - Classes and Objects
Read the following in Just Java 2:
- Chapter 2: Introducing Objects
- Chapter 3: Primitive Types, Wrappers, and Boxing
- Chapter 5: OOP Part II -- Constructors and Visibility
- Chapter 8: More OOP -- Extending Classes.
- Chapter 10: Exceptions
Or, if you have the older edition (Just Java 1.2), read chapters 2, 3, 6 and the last half of chapter 7.
Chapter 2 introduces object oriented programming and how it is used in
Java. Read this chapter thoroughly. Maybe read it twice. The stuff
in this chapter and in chapter 6 is 90% of the questions that will be
asked during most Java tech interviews. (I don't think this is what
should be asked during a Java tech interview, but I'll save that rant
for another time.)
Chapter 3 walks you through a sample program and how that program is
run in the Java Virtual Machine (JVM). This jumps ahead of us a
little bit by using the AWT (Abstract Windowing Toolkit), but it's
easy to follow. Keep in mind that the author is one of the JVM kernel
developers - so this information is right from the horse's mouth!
Chapter 5 and 8 are going to introduce you to inheritance. More primary
interview material. Inheritance is spiffy and easy. Some people have
trouble with the idea of overriding a method and the "abstract"
keyword. If you have trouble, be sure to ask on the Java College
forum. You will want to understand it well now. Just browse the last
part of chapter 8 ("The Class Whose Name Is Class") on reflection.
You might want to try and do the exercises at the end of the chapter.
Chapter 10 is about exceptions and error handling.
Read "Cup Size", "Pass-by-value Please" and "How my Dog learned Polymorphism" at the JavaRanch Campfire.
Assignment OOP-1 (DaysOld)
Purpose: To learn how to use someone else's classes, how to integrate a jar file into your program
Write a program that tells you how many days old you are.
See www.javaranch.com/common.jsp
and look for the GDate and JDate classes.
Creating objects from these classes will help you to complete this assignment.
You will need to download jr.jar and work it into your classpath
in order to use these classes.
In other words, I want to type
java DaysOld 2000-1-2
and see
You were born on January 2, 2000
Today is November 18, 2000
You are now 321 days old.
(with the correct information for the dates and number of days)
To get this to work, you must properly configure your classpath. If you write all
of these programs in the C:\Java directory, you could put the .jar file in the same
directory and change your classpath to "C:\Java;C:\Java\jr.jar".
Be sure to put "import com.javaranch.common.* ;" at the top of your DaysOld.java
file.
Instructor's solution:
Assignment OOP-2 (NaturalLanguageMultiply)
Purpose: To learn how to work with a HashMap, how to use static blocks to initialize,
how to throw and catch exceptions
Write a program that will multiply two numbers in the range of
zero to ninety-nine.
In other words, I want to type
java NaturalLanguageMultiply thirty-two ten
and see
320
Notes:
It is okay to have global constants in a program. You can
initialize global constants with a static block if you need to.
Example of a static block:
public class Test
{
private static Vector vector ;
static
{
vector = new Vector();
vector.add( "foo" );
vector.add( "bar" );
}
}
If you create a method or class attribute that is for internal use only be sure to
declare it private.
Use one HashMap object that contains no more than 28 objects.
Include a method with this signature: "private static int toInt( String s ) throws Exception".
The exception is if the string is not recognized and contains the message you want to show to your user.
The program must specify what text is not recognized.
Instructor's solution:
Assignment OOP-3 (SortNames)
Purpose: To learn how to work with an ArrayList, how to implement an interface, and how to use I/O
Load an ArrayList with Strings from a text file of names. Show the names sorted in order of first name and then by last name. Use com.javaranch.common.TextFileIn to read the names. Use the Collections class for sorting. Do not create a second list with the names in a different order. Do not modify the ArrayList or its contents except by using the Collections class. Your class will be called SortNames.
Here is the list of names .
Instructor's solution:
Assignment OOP-4 (Lookup)
Purpose: To learn about polymorphism
Rudy's Rental rents all sorts of stuff: videos, books on tape, furniture,
etc. Every thing that Rudy rents has a serial number. Write a program
called Lookup that takes in a serial number and writes information on a
given item to the console. You will need to make an abstract class
called Thing and three subclasses: Video, BookOnTape and Furniture. There
will be an abstract method called getDescription() that returns a String.
Here is a UML diagram showing the three sub classes inheriting Thing.
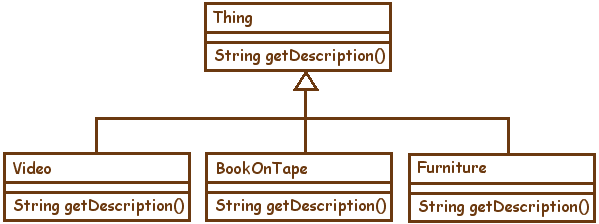
Lookup will contain a static HashMap that contains a dozen things to rent.
Make up whatever things you like as long as you use all three types. Give
each of your classes relevant attributes and have the getDescription()
method return as much information on an item as possible.
Instructor's solution
After you have tied up this class, lasso the next one:
Page maintained by
Marilyn de Queiroz
|